CSE Helper
Powered By Dream Dragon Creation
Doubly Linked List
Continue of Single Linked List . . .
The two node links allow traversal of the list in either direction. While adding or removing a node in a doubly-linked list requires changing more links than the same operations on a singly linked list, the operations are simpler and potentially more efficient (for nodes other than first nodes) because there is no need to keep track of the previous node during traversal or no need to traverse the list to find the previous node, so that its link can be modified.
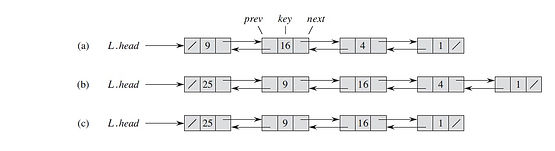
DSome operation in Doubly Linked list. most of the operations same as single Linked list..
Insert funtion
LIST-INSERT( L , x )
1 x.next = L.next
2 If ( L.head != Null )
3 L.head.prev = x
4 L.head = x
5 x.prev = null
Search Funtion
LIST-SEARCH (L , k )
1 x = L.head
2 while x != NIL and x.key != k
3 x = x.next
4 return x
Delete funtion
LIST-DELETE (L , x )
1 if x.pre != NIL
2 x.pre.next = x.next
3 else L.head = x.next
4 if x.next != NIL
5 x.next.pre = x.pre
Doubly Linked List implementation in C
1. /* Doubly Linked List implementation */
2. #include<stdio.h>
3. #include<stdlib.h>
4. struct Node {
5. ........int data;
6. ........struct Node* next;
7. ........struct Node* prev;
8. };
9. struct Node* head; // global variable - pointer to head node.
10. int length; // global variable - length of list
11. void init_list(){
12. ........head = nullptr;
13. ........length = 0;
14. }
15. bool is_list_empty(){
16. ........if (length == 0){
17. ..................return true;
18. ........}
19. ........return false;
20. }
21. struct Node* search(int element){
22. ........struct Node* newNode = head;
23. ........while (newNode != nullptr)
24. ........{
25. ................if (newNode->data == element){
26. .........................return newNode;
27. ................}
28. ................newNode = newNode->next;
29. .......}
30. }
31. bool deleteNode( int element){
32. ........struct Node* delNode = search(element);
33. ........if (delNode != NULL){
34. ................delNode->prev->next = delNode->next;
35. ................delete delNode;
36. ................return true;
37. ........}
38. ........else{
39. ................printf(" element not found! \n");
40. ................return false;
41. .......}
42. }
43. bool deleteat( int i){
44. ........if (i < length){
45. ................struct Node* newNode = head;
46. ................for (int j = 0; j < i; j++){
47. ......................newNode = newNode->next;
48. ................}
49. ................newNode->prev->next = newNode->next;
50. ................delete newNode;
51. ................return true;
52. .......}
53. ........printf(" List index not valid!.");
54. }
55. //Inserts a Node at tail of Doubly linked list
56. int insert(int element){
57. ........struct Node* temp = head;
58. ........struct Node* newNode
59. ................= (struct Node*)malloc(sizeof(struct Node));
60. ........newNode->data = element;
61. ........if (head == NULL) {
62. ................head = newNode;
63. ................return 1;
64. ........}
65. ........while (temp->next != NULL) temp = temp->next; // Go To last Node
66. ........temp->next = newNode;
67. ........newNode->prev = temp;
68. }
69. int insertat(int element, int i){
70. ........if (i < length){
71. ................struct Node* temp = head;
72. ................struct Node* newNode
73. ...........................= (struct Node*)malloc(sizeof(struct Node));
74. ................newNode->data = element;
75. ................if (head == NULL) {
76. ........................head = newNode;
77. ........................return 1;
78. ................}
79. ................int j = 0; // go to given index
80. ................while (j < i) {
81. ................temp = temp->next;
82. .............................j++;
83. ................}
84. ................// insert after given index
85. ................newNode->next = temp->next;
86. ................temp->next = newNode;
87. ................newNode->prev = temp;
88. .......}
89. }
90. int list_length(){
91. .........return length;
92. }
93. //Prints all the elements in linked list in forward traversal order
94. void Print() {
95. ........struct Node* temp = head;
96. ........printf("Forward: ");
97. ........while (temp != NULL) {
98. ................printf("%d ", temp->data);
99. ................temp = temp->next;
100. ........}
101. ........printf("\n");
102. }
103. //Prints all elements in linked list in reverse traversal order.
104. void ReversePrint() {
105. ........struct Node* temp = head;
106. ........if (temp == NULL) return; // empty list, exit
107. ........// Going to last Node
108. ........while (temp->next != NULL) {
109. ..............temp = temp->next;
110. ........}
111. .........// Traversing backward using prev pointer
112. ........printf("Reverse: ");
113. ........while (temp != NULL) {
114. ................printf("%d ", temp->data);
115. ................temp = temp->prev;
116. ........}
117. ........printf("\n");
118. }
119. int main() {
120. ......../*Driver code to test the implementation*/
121. ........head = NULL; // empty list. set head as NULL.
122. ........// Calling an Insert and printing list both in forward as well as reverse direction.
123. ........insert(2); Print(); ReversePrint();
124. ........insert(4); Print(); ReversePrint();
125. ........insert(6); Print(); ReversePrint();
126. ........insertat(8,2); Print(); ReversePrint();
127. ........system("PAUSE");
128. }
Doubly Linked List – C++ Program source code
-
#include <iostream>
-
-
using namespace std;
-
typedef int E;
-
-
class Node {
-
private:
-
E value;
-
Node* next;
-
Node* prev;
-
public:
-
E Node::getValue();
-
void Node::setValue(E val);
-
Node* Node::getNext();
-
void Node::setNext(Node* newNode);
-
Node* Node::getprev();
-
void Node::setprev(Node* newNode);
-
};
-
class LinkedList {
-
-
private:
-
Node* head;
-
int length;
-
-
public:
-
void LinkedList::init_list();
-
bool LinkedList::is_list_empty();
-
bool LinkedList::search(E value);
-
E LinkedList::deleteNode(E value);
-
E LinkedList::deleteat(int i);
-
bool LinkedList::insert(E value);
-
bool LinkedList::insertat(E value, int i);
-
int LinkedList::list_length();
-
};
-
-
using namespace std;
-
-
E Node::getValue(){
-
return this->value;
-
}
-
void Node::setValue(E val){
-
this->value = val;
-
}
-
Node* Node::getNext(){
-
return this->next;
-
}
-
void Node::setNext(Node* newNode){
-
this->next = newNode;
-
}
-
Node* Node::getprev(){
-
return this->prev;
-
}
-
void Node::setprev(Node* newNode){
-
this->prev = newNode;
-
}
-
-
using std::cout;
-
using std::endl;
-
// declaring the linked list
-
void LinkedList::init_list(){
-
head = NULL;
-
length = 1;
-
}
-
-
//cheak whether linked list is empty
-
bool LinkedList::is_list_empty(){
-
if (head == NULL){
-
return true;
-
}
-
return false;
-
}
-
-
// searching value in the linked list
-
bool LinkedList::search(E value){
-
Node* p = head;
-
// declaring the pointer to travel thought linked list
-
if (is_list_empty()) // cheaking list is emply
-
{
-
cout << "List is empty" << endl;
-
return false;
-
}
-
while (p != NULL)
-
{ //conditional statement
-
if (p->getValue() == value){
-
cout << "The value is in the list" << endl;
-
return true;
-
}
-
p = p->getNext();
-
}
-
cout << "value not in the list" << endl;
-
-
}
-
-
// deleting value in given location
-
E LinkedList::deleteat(int i){
-
Node* p = head;
-
int l = list_length();
-
if (l < i){
-
cout << "location not exist" << endl;
-
return 0;
-
}
-
while (l>i - 1){
-
p = p->getNext();
-
}
-
Node* del = p->getNext();
-
p->setNext(del->getNext());
-
cout << i << " th item deleted" << endl;
-
return 1;
-
}
-
-
//insert the value to list at tail
-
bool LinkedList::insert(E value){
-
Node* temp = head;
-
Node* newNode = new Node;
-
newNode->setvalue(value);
-
if( head == NULL){
-
head = newNode;
-
return 1;
-
}
-
while(temp->getNext() != NULL){
-
temp = temp->getNext; // Go to last node
-
}
-
temp->setNext(newNode);
-
newNode->setprev(temp);
-
}
-
-
-
//insert the value to the given location
-
bool LinkedList::insertat(E value, int i){
-
Node* p = head;
-
if (list_length() < i){
-
cout << "location not exist" << endl;
-
return false;
-
}
-
int l = list_length();
-
// travel to the given location
-
while (l>i){
-
p = p->getNext();
-
}
-
// craeting new node to set values
-
Node* N_node = new Node;
-
N_node->setValue(value);// setting value
-
N_node->setNext(p->getNext());// setting next pointer
-
p->setNext(N_node); // setting previous pointer
-
N_node->setprev(p);
-
length++; // increament the lenght of list
-
return true;
-
-
}
-
-
//return the length of the linked list
-
int LinkedList::list_length(){
-
return length;
-
}
-
-
void main(int argc, char **argv)
-
{
-
/* Create an empty list */
-
LinkedList list1;
-
list1 init_list();
-
cout << "Created an empty list named list1 " << endl;
-
// inserting elements to the list
-
if (list1 insert(10)){
-
cout << "Insert : 10" << endl;
-
}
-
if (list1 insert(24)){
-
cout << "Insert : 24" << endl;
-
}
-
if (list1 insert(65)){
-
cout << "Insert : 65" << endl;
-
}
-
cout << "search 35 in the list" << endl;
-
list1 search(35);
-
cout << "length of list :" << list1 list_length() << endl;
-
cout << "delete 2nd element" << endl;
-
list1 deleteat(2);
-
}
Doubly Linked List Implementation in Java
-
package doubly_Lined_List;
-
/**
-
*
-
@author cse helper
-
-
*/
-
public class Node {
-
private Node next;
-
private Node back;
-
private int value;
-
-
public void setValue ( int value ) {
-
this.value = value;
-
}
-
public void setnext ( Node node ) {
-
next = node;
-
}
-
public void setback ( Node node ) {
-
back = node;
-
}
-
public int getValue ( ) {
-
return value;
-
}
-
public Node getnext(){
-
return next;
-
}
-
public Node getback(){
-
return back;
-
}
-
}
-
-
public class Doubly_Linked_List {
-
private Node head;
-
private int length;
-
-
public void init_list ( ) {
-
head = null;
-
length = 0;
-
}
-
public boolean is_empty_list(){
-
if ( head == null ) {
-
return true;
-
}
-
return false;
-
}
-
public boolean search (int value ) {
-
Node p = head;
-
if ( is_empty_list ( ) ) {
-
System.out.println("List is empty");
-
return false;
-
}
-
while(p != null){
-
if ( p.getValue ( ) == value ) {
-
System.out.println("The value is in the list");
-
return true;
-
}
-
p = p.getnext ( ) ;
-
}
-
System.out.println("value not in the list");
-
return false;
-
}
-
public boolean insert ( int value){
-
Node temp = head;
-
Node newNode = new Node( ) ;
-
newNode.setValue ( value ) ;
-
if ( head == null ) {
-
head = newNode;
-
length++;
-
return true;
-
}else{
-
while ( temp.getnext ( ) != null ) {
-
temp = temp.getnext ( ) ;
-
}
-
temp.setnext ( newNode ) ;
-
newNode.setback ( temp ) ;
-
length ++;
-
return true;
-
}
-
}
-
public boolean inserAt ( int value ,int i ) {
-
Node p = head;
-
if ( list_length ( ) < i ) {
-
System.out.println ( " location not exist");
-
return false;
-
}
-
int l = list_length ( ) ;
-
//travel to given location
-
while ( l > i - 1 ) {
-
p = p.getnext ( ) ;
-
}//creating new node to set values
-
Node newNode = new Node ( ) ;
-
newNode.setValue ( value ) ; // setting value
-
newNode.setnext( p.getnext ( ) ) ;
-
p.setnext ( newNode ) ; // setting previous node
-
newNode.setback ( p ) ;
-
length++;
-
return true;
-
}
-
public boolean delete( int value ) {
-
Node p = head;
-
if ( is_empty_list ( ) ) {
-
System.out.println ( "List is empty " ) ;
-
return false;
-
}
-
// if value in head deleted
-
if ( head.getValue ( ) == value ) {
-
head = head.getnext ( ) ;
-
head.setback ( null ) ;
-
length- -;
-
return true;
-
}
-
// delete other node
-
while ( p != null) {
-
if ( p.getValue ( ) == value ){
-
p.getback( ).setnext ( p.getnext ( ) ) ;
-
p.getnext( ).setback ( p.getback ( ) );
-
System.out.println("The value is deleted");
-
length- - ;
-
return true;
-
} p = p.getnext();
-
}
-
System.out.println ( "value not in the list");
-
return false;
-
}
-
public boolean deleteAt ( int i ) {
-
Node p = head;
-
int l = list_length ( ) ;
-
if ( l < i ) {
-
System.out.println ( "Location not found ");
-
return false;
-
}
-
while ( l > ( i - 1 ) ) {
-
p = p.getnext ( ) ;
-
}
-
Node del = p.getnext ( ) ;
-
p.setnext ( del.getnext ( ) ) ;
-
System.out.println ( String valueOf ( i ) +" th item deleted ");
-
length --;
-
return true;
-
}
-
public int list_length(){
-
return length;
-
}
-
public void printList(){
-
Node print = head;
-
while(print != null){
-
System.out.print(print getValue());
-
System.out.print(" ");
-
print = print. getnxt( );
-
}
-
System.out.println("");
-
}
-
-
public static void main( String[ ] args){
-
Doubly_Linked_List list1 = new Doubly_Linked_List();
-
list.init_list();
-
list1.insert(10);
-
list1.insert(20);
-
list1.printList();
-
list1. insert(15);
-
list1.printList();
-
list1.inserAt(45, 1);
-
list1.insert(36);
-
list1.delete(20);
-
list1.delete(6);
-
list1. search(14);
-
list1 deleteAt(2);
-
}
-
}